# Skyboxes
All API endpoints require an API Key sent in a header or as a get parameter. Please refer to the Making requests section to find out more.
# Generate Skybox
This section explains how to generate skyboxes.
To generate a skybox, start by selecting a skybox style. You'll need to use the id
of the chosen style as the skybox_style_id
parameter in your request. For more details on how to retrieve available styles, refer to the Get Skybox Styles section.
# Method
POST
# API Endpoint
https:/api/v1/skybox
# Params
Key | Data Type | Description | |
---|---|---|---|
skybox_style_id | Int | Predefined styles are used to choose the model version and influence the overall aesthetic of your skybox generation (Fantasy landscape, Anime art style, etc...). Explained in more detail here. | required |
prompt | String | Text prompt describing the skybox world you wish to create. Maximum number of characters: 2000 . If you are using skybox_style_id then the maximum number of characters is defined in the max-char response parameter defined for each style | required |
negative_text | String | Describe things to avoid in the skybox world you wish to create. Maximum number of characters: 1000 . If you are using skybox_style_id then the maximum number of characters is defined in the negative-text-max-char response parameter defined for each style | optional |
enhance_prompt | Boolean | Have an AI automatically improve your prompt to generate pro-level results every time (default: false ) | optional |
seed | Int | Send 0 for a random seed generation. Any other number (1-2147483647) set will be used to "freeze" the image generator and create similar images when run again with the same seed and settings. | optional |
remix_imagine_id | Int | ID of a previously generated skybox. | optional |
control_image | Binary or Base64 string or Absolute URL | Control image used to influence the generation (ie. remix from init image). Explained in more detail here. | optional |
control_model | String | Model used for the control_image . Currently the only option is: "remix" . | optional |
webhook_url | String | Optionally, you may specify a webhook url to specify the destination for progress updates | optional |
# Generate skybox request example
To generate a skybox send application/json
POST request to https://backend.blockadelabs.com/api/v1/skybox
with prompt
parameter as a description of the skybox world you wish to create. You can also try our Postman collection to start testing:
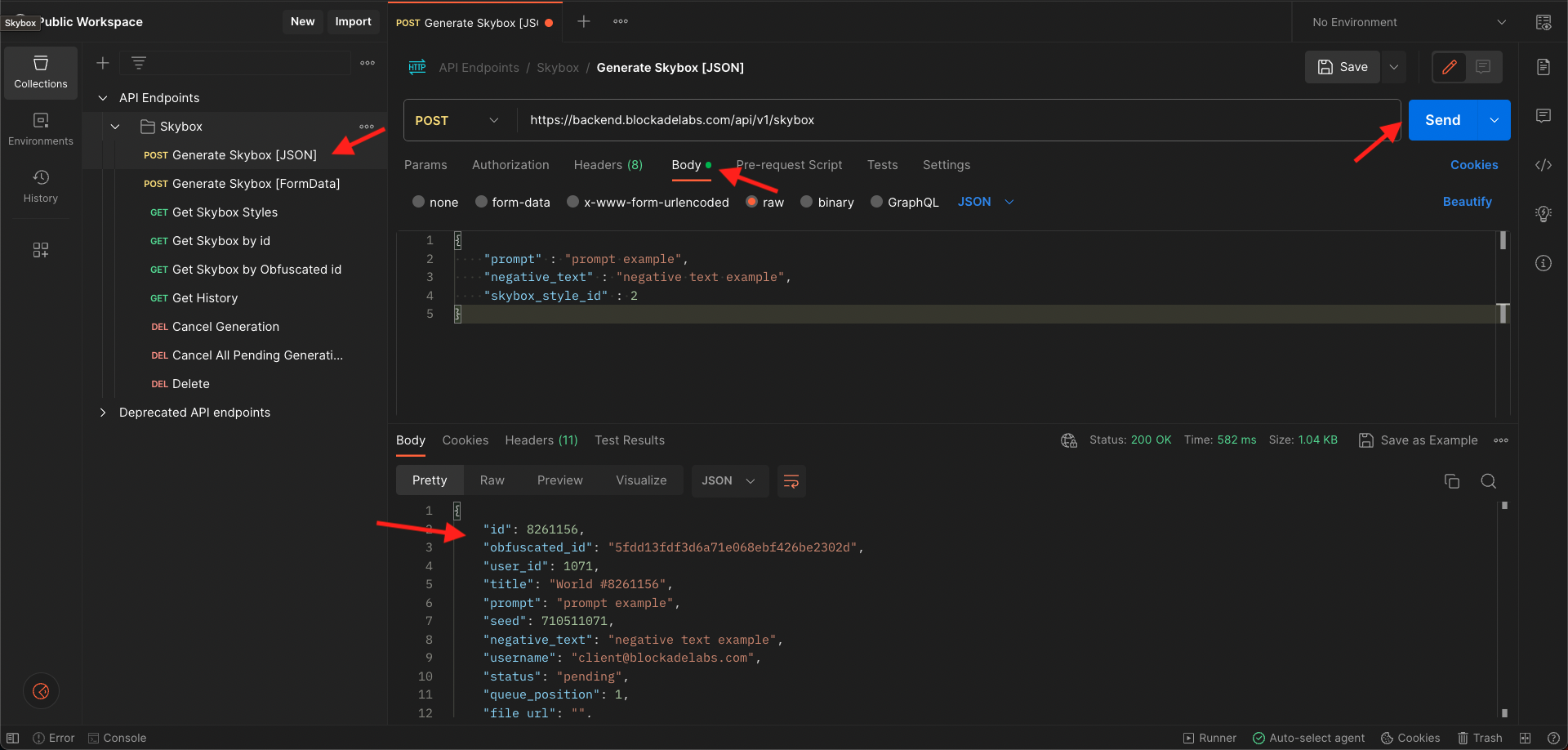
# Response example
{
"id": 123456, // id of your generation. It can be used to track generation progress or to cancel the generation
"skybox_style_id": 67, // skybox style id used in a generation request
"skybox_style_name": "M3 Photoreal", //skybox style name used in a generation request
"model": "Model 3", // model used to generate
"status": "pending", // initially status is set as 'pending' after you send a generation request. Status will change from one state to another and you will get updates for each one if you are using pusher or webhook: pending -> dispatched -> processing -> complete. Also you can get abort or error.
"type": "skybox", // type of skybox generation
"queue_position": 2, // position of your request in a generation queue
"file_url": "", // full size of generated image url (empty until generation is completed)
"thumb_url": "", // thumbnail of generated image url (empty until generation is completed)
"title": "Imagination #123456", // generation title
"user_id": 1, // your user id
"username": "user@blockadelabs.com", // your username
"error_message": null, // if status=error here you should see the error message
"obfuscated_id": "460370b7328a5cb8dbddd6ef0d1c9dd4", // hash id of your generation
"pusher_channel": "status_update_460370b7328a5cb8dbddd6ef0d1c9dd4", // pusher channel name used to track generation progress
"pusher_event": "status_update", // pusher channel event used to track generation progress
"created_at": "2023-03-18T10:42:19+00:00", // time created
"updated_at": "2023-03-18T10:42:19+00:00" // time updated
}
TIP
Don't forget that after you receive the response above you need to track generation progress.
# Generation progress - Status update message example:
{
"id": 123456,
"obfuscated_id": "460370b7328a5cb8dbddd6ef0d1c9dd4",
"skybox_style_id": 67,
"skybox_style_name": "M3 Photoreal",
"model": "Model 3",
"status": "complete",
"queue_position": 1,
"file_url": "https://blockade-platform-production.s3.amazonaws.com/images/imagine/detailed_digital_painting_cd_computer_render_fantasy_vr_dreamscape__6fee994c6f9f2229__793071_6.jpg?ver=1",
"thumb_url": "https://blockade-platform-production.s3.amazonaws.com/thumbs/imagine/thumb_detailed_digital_painting_cd_computer_render_fantasy_vr_dreamscape__6fee994c6f9f2229__793071_6.jpg?ver=1",
"depth_map_url": "https://blockade-platform-production.s3.amazonaws.com/depths/imagine/detailed_digital_painting_cd_computer_render_fantasy_vr_dreamscape__6fee994c6f9f2229__793071_6.jpg?ver=1",
"title": "Imagination #123456",
"user_id": 1,
"username": "user@blockadelabs.com",
"error_message": null,
"pusher_channel": "status_update_460370b7328a5cb8dbddd6ef0d1c9dd4",
"pusher_event": "status_update",
"created_at": "2023-03-18T10:42:19+00:00",
"updated_at": "2023-03-18T10:42:36+00:00"
}
# Generate Skybox Examples
Use the following examples as a request parameters for the generate skybox API request:
POST https://backend.blockadelabs.com/api/v1/skybox
# Generate Skybox using the "M3 UHD Render" style
{
"skybox_style_id": 35, // "M3 UHD Render" skybox style id
"prompt": "prompt example",
}
# Generate Skybox using the "M3 UHD Render" style, with negative text, seed, prompt enhancer and webhook_url
{
"skybox_style_id": 35, // "M3 UHD Render" skybox style id
"prompt": "mountains", // when enhance_prompt is sent this text will be used by AI to improve it
"negative_text": "clouds, river", // avoid generating clouds and river
"enhance_prompt": true, // automatically improve the prompt
"seed": 234143, // set generation seed number
"webhook_url": "https://www.example.com/webhook", // the url destination where the progress updates should be sent
}
# Remix previously generated skybox
{
"skybox_style_id": 35, // "M3 UHD Render" skybox style id
"prompt": "prompt example",
"remix_imagine_id": 123456 // id of a previously generated skybox
}
# Remix from uploaded (equirectangular) image
{
"skybox_style_id": 35, // "M3 UHD Render" skybox style id
"prompt": "prompt example",
"control_image": "https://images.blockadelabs.com/skybox-starters/42_133ff00e73def7ca30c01b24be7991d9_file.jpg", // this parameter can be sent as Binary, Base64 string or Absolute URL string
"control_model": "remix" // required parameter in order for "remix from uploaded image" to work
}
# Best practices
The control_image
should be an equirectangular image sent across as binary, base64 or absolute URL with a common resolution of 2048x1024 or greater, with an aspect ratio of 2:1 and a max file size of 50MB. Images submitted outside of the aspect ratio of 2:1 will be forced to a 2:1 ratio during processing which could produce undesired image warping.
# Get Skybox Styles
This endpoint returns a list of predefined styles that shape the overall aesthetic of your skybox generation.
Each style in the response includes model
and model_version
parameters, indicating the model that will be used for generation.
Since Model 2
is now deprecated, ensure you request this endpoint with the model_version
parameter set to 3
.
For example:
https://backend.blockadelabs.com/api/v1/skybox/styles?model_version=3
.
# Method
GET
# API Endpoint
https:/api/v1/skybox/styles
# Params
Key | Data Type | Description | |
---|---|---|---|
model_version | String | Filter skybox styles by model version number. Since model 2 is now deprecated only available option is 3 . | optional |
# Response example
[
{
"id": 67, // to be used as 'skybox_style_id' parameter in the 'Generate skybox' endpoint to influence the overall aesthetic of your skybox generation
"name": "M3 Photoreal", // name of the style
"max-char": 390, // maximum number of characters that can be used for 'prompt' in the 'Generate skybox' endpoint
"negative-text-max-char": 250, // maximum number of characters that can be used for 'negative_text' in the 'Generate skybox' endpoint
"image": "https://blockade-platform-production.s3.amazonaws.com/thumbs/imagine/thumb_detailed_digital_painting_cd_computer_render_fantasy_vr_dreamscape__6fee994c6f9f2229__793071_6.jpg", // image example of the style
"sort_order": 1, // sorting order
"model": "Model 3", // model name
"model_version": "3" // model version number
},
{
"id": 43,
"name": "Anime art style (Model 3)",
"max-char": 360,
"negative-text-max-char": 220,
"image": "https://blockade-platform-production.s3.amazonaws.com/thumbs/imagine/thumb_beautiful_anime_illustration_vr_view_anime_cinematic_lighting__d8f7004caba3e61c__863995_d8f700.jpg",
"sort_order": 2,
"model": "Model 3",
"model_version": "3"
},
...
]
# Get Skybox by id
# Method
GET
# API Endpoint
https:/api/v1/imagine/requests/{id}
# Response example
{
"request" : {
"id": 123456, // id of your generation. It can be used to track generation progress or to cancel the generation
"obfuscated_id": "460370b7328a5cb8dbddd6ef0d1c9dd4", // hash id of your generation
"user_id": 1, // your user id
"username": "user@blockadelabs.com", // your username
"status": "complete", // initially status is set as 'pending' after you send a generation request. Status will change from one state to another and you will get updates for each one if you are using pusher or webhook: pending -> dispatched -> processing -> complete. Also you can get abort or error.
"queue_position": 1, // position of your request in a generation queue
"pusher_channel": "status_update_460370b7328a5cb8dbddd6ef0d1c9dd4", // pusher channel name used to track generation progress
"pusher_event": "status_update", // pusher channel event used to track generation progress
"error_message": null, // if status=error here you should find the error message
"type": "skybox", // type of generation (currently "skybox" is the only one)
"title": "Imagination #123456", // generation title
"prompt": "prompt text", // prompt text used to generate skybox
"seed": 123456, // seed number used to generate skybox
"skybox_style_id": 67, // skybox style id used to generate skybox
"skybox_style_name": "M3 Photoreal", // skybox style name used to generate skybox
"model": "Model 3", // model name used to generate skybox
"file_url": "https://blockade-platform-production.s3.amazonaws.com/images/imagine/futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox image (6144x3072 pixels)
"thumb_url": "https://blockade-platform-production.s3.amazonaws.com/thumbs/imagine/thumb_futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox thumbnail (672x336 pixels)
"depth_map_url": "https://blockade-platform-production.s3.amazonaws.com/depths/imagine/futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox depyh map image (2048x1024 pixels)
"created_at": "2023-03-18T10:42:19+00:00", // time created
"updated_at": "2023-03-18T10:42:39+00:00", // time updated
"dispatched_at": "2023-03-18T10:42:20+00:00", // time dispatched
"processing_at": "2023-03-18T10:42:21+00:00", // time processing started
"completed_at": "2023-03-18T10:42:39+00:00", // time completed
}
}
# Get Skybox by Obfuscated id
# Method
GET
# API Endpoint
https:/api/v1/imagine/requests/obfuscated-id/{obfuscatedId}
# Response example
{
"request" : {
"id": 123456, // id of your generation. It can be used to track generation progress or to cancel the generation
"obfuscated_id": "460370b7328a5cb8dbddd6ef0d1c9dd4", // hash id of your generation
"user_id": 1, // your user id
"username": "user@blockadelabs.com", // your username
"status": "complete", // initially status is set as 'pending' after you send a generation request. Status will change from one state to another and you will get updates for each one if you are using pusher or webhook: pending -> dispatched -> processing -> complete. Also you can get abort or error.
"queue_position": 1, // position of your request in a generation queue
"pusher_channel": "status_update_460370b7328a5cb8dbddd6ef0d1c9dd4", // pusher channel name used to track generation progress
"pusher_event": "status_update", // pusher channel event used to track generation progress
"error_message": null, // if status=error here you should find the error message
"type": "skybox", // type of generation (currently "skybox" is the only one)
"title": "Imagination #123456", // generation title
"prompt": "prompt text", // prompt text used to generate skybox
"seed": 123456, // seed number used to generate skybox
"skybox_style_id": 67, // skybox style id used to generate skybox
"skybox_style_name": "M3 Photoreal", // skybox style name used to generate skybox
"model": "Model 3", // model name used to generate skybox
"file_url": "https://blockade-platform-production.s3.amazonaws.com/images/imagine/futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox image (6144x3072 pixels)
"thumb_url": "https://blockade-platform-production.s3.amazonaws.com/thumbs/imagine/thumb_futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox thumbnail (672x336 pixels)
"depth_map_url": "https://blockade-platform-production.s3.amazonaws.com/depths/imagine/futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox depyh map image (2048x1024 pixels)
"created_at": "2023-03-18T10:42:19+00:00", // time created
"updated_at": "2023-03-18T10:42:39+00:00", // time updated
"dispatched_at": "2023-03-18T10:42:20+00:00", // time dispatched
"processing_at": "2023-03-18T10:42:21+00:00", // time processing started
"completed_at": "2023-03-18T10:42:39+00:00", // time completed
}
}
# Get History
# Method
GET
# API Endpoint
https:/api/v1/imagine/myRequests
# Params
Key | Data Type | Description | |
---|---|---|---|
status | String | Filter by status. Options: all, pending, dispatched, processing, complete, abort, error (default: all) | optional |
limit | Int | Number of items to be returned per page (default: 18, max-value: 100) | optional |
offset | Int | Page number (default: 0) | optional |
order | String | Sort order. Options: ASC, DESC (default: DESC) | optional |
imagine_id | Int | Filter by skybox id | optional |
query | String | Filter by title or prompt | optional |
generator | String | Filter by generator | optional |
# Response example
{
"data": [
{
"id": 123456, // id of your generation. It can be used to track generation progress or to cancel the generation
"obfuscated_id": "460370b7328a5cb8dbddd6ef0d1c9dd4", // hash id of your generation
"user_id": 1, // your user id
"username": "user@blockadelabs.com", // your username
"status": "complete", // initially status is set as 'pending' after you send a generation request. Status will change from one state to another and you will get updates for each one if you are using pusher or webhook: pending -> dispatched -> processing -> complete. Also you can get abort or error.
"queue_position": 1, // position of your request in a generation queue
"pusher_channel": "status_update_460370b7328a5cb8dbddd6ef0d1c9dd4", // pusher channel name used to track generation progress
"pusher_event": "status_update", // pusher channel event used to track generation progress
"error_message": null, // if status=error here you should find the error message
"type": "skybox", // type of generation (currently "skybox" is the only one)
"title": "Imagination #123456", // generation title
"prompt": "prompt text", // prompt text used to generate skybox
"seed": 123456, // seed number used to generate skybox
"skybox_style_id": 67, // skybox style id used to generate skybox
"skybox_style_name": "M3 Photoreal", // skybox style name used to generate skybox
"model": "Model 3", // model name used to generate skybox
"file_url": "https://blockade-platform-production.s3.amazonaws.com/images/imagine/futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox image (6144x3072 pixels)
"thumb_url": "https://blockade-platform-production.s3.amazonaws.com/thumbs/imagine/thumb_futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox thumbnail (672x336 pixels)
"depth_map_url": "https://blockade-platform-production.s3.amazonaws.com/depths/imagine/futuristic_microdetailed_vr_scifi_concept_art_cinematic_vr_neon__dbe7f963dc23699c__2757929_dbe7.jpg?ver=1", // generated skybox depyh map image (2048x1024 pixels)
"created_at": "2023-03-18T10:42:19+00:00", // time created
"updated_at": "2023-03-18T10:42:39+00:00", // time updated
"dispatched_at": "2023-03-18T10:42:20+00:00", // time dispatched
"processing_at": "2023-03-18T10:42:21+00:00", // time processing started
"completed_at": "2023-03-18T10:42:39+00:00", // time completed
}
],
"totalCount": 1,
"has_more": false
}
# Cancel Generation
# Method
DELETE
# API Endpoint
https:/api/v1/imagine/requests/{id}
TIP
This request sets the status to the abort
value.
# Response example
{
"success": true
}
# Cancel All Pending Generations
This request sets the status of all pending
generations to abort
.
# Method
DELETE
# API Endpoint
https:/api/v1/imagine/requests/pending
# Response example
{
"success": true
}
# Delete
This request deletes skybox.
# Method
DELETE
# API Endpoint
https:/api/v1/imagine/deleteImagine/{id}
# Response example
{
"success": "Item deleted successfully",
"id": "42"
}